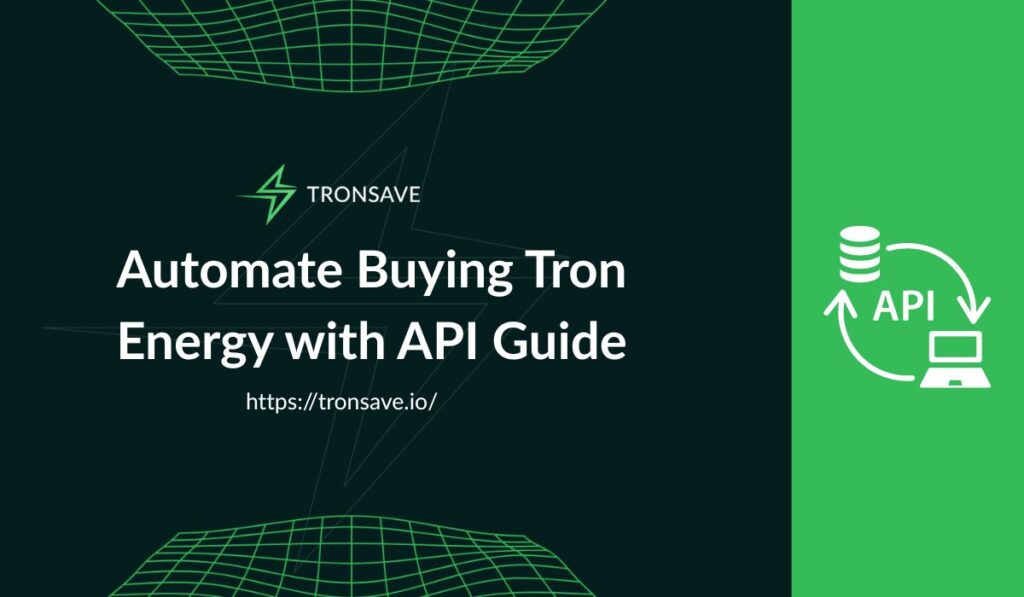
KEY TAKEAWAYS
- Slash USDT TRC20 transaction fees by 80-90% by automating Tron energy purchases via API.
- Use Tronsave’s API to buy energy instantly, saving $100s monthly on TRC20 gas fees.
- Set up in 10 minutes with our step-by-step code, plus batch purchases for dApps.
Why Tron Energy Matters (and Why You’re Losing Money Without It)
Picture Tron’s blockchain as a highway. Bandwidth is your free pass for simple TRX transfers, with 600 points daily (enough for ~2 sends). But Tron energy? That’s the rocket fuel for smart contracts, like USDT TRC20 transfers or DeFi swaps. Without it, you’re burning TRX—big time.
Here’s the kicker: a USDT transfer needs 64,285 energy units. No energy? You’ll burn ~13.5 TRX ($3.37 at $0.25/TRX). Buy Tron energy with API, and it’s just 3 TRX ($0.75)—an 80% savings. I saw a DeFi dApp drop monthly USDT TRC20 transaction fees from $500 to $60 by automating with Tronsave. That’s real money back in your pocket.
Why automate? A Tron energy API lets you:
- Scale fast: Handle 1,000s of transactions without lifting a finger.
- Save time: No more manual energy refills.
- Boost dApps: Embed purchases for seamless user experiences.
What most folks miss: automation isn’t just convenient – it’s a game-changer. So, how much could you save by automating your dApp’s energy? Let’s find out.
Picking the Right Tron Energy Provider
Not all Tron energy markets are built the same, and a wrong choice can mean flaky APIs or hidden fees. I learned this the hard way when a provider’s spotty docs cost me 50 TRX in failed tests. With options like Tronsave, Tron Energy Market, and TronEnergize, here’s how to choose wisely in the Tron energy exchange.
Look for:
- Cost per unit: Tronsave offers from 0.00005 TRX/energy.
- API reliability: 99.9% uptime is a must – check reviews on r/Tronix.
- Docs quality: Clear Tron API documentation (like Tronsave’s at docs.tronsave.io) saves hours.
- Testnet support: Test on Nile to avoid burning TRX.
Pro tip: Don’t bet on one provider. If their API crashes, your dApp stalls. Test two and add failover logic. Grab our free “Provider Comparison Checklist” to pick like a pro.
Table: Energy Provider Showdown
Provider | Price (TRX/energy) | Docs Quality | Testnet |
Tronsave | 0.00005 | Excellent | Yes |
Tron Energy Market | 0.000045 | Great | Yes |
TronEnergize | 0.000045 | Poor | No |
Setting Up Your API Environment
Time to get hands-on. Setting up to buy Tron energy with API is straightforward, but one misstep can burn TRX. I fumbled Node.js configs at 2 a.m. once—don’t be me. Here’s a foolproof setup with Tronsave’s API, built for security and scale.
Step 1: Install Node.js and TronWeb
- Grab Node.js (v16+) from nodejs.org. Check with node -v.
- Install TronWeb:
bash
npm install tronweb
- Verify: npm list tronweb (should show [email protected]).
Step 2: Secure Your Keys
- Get your API key from docs.tronsave.io/developer/get-api-key.
- Store private and API keys safely:
bash
echo “PRIVATE_KEY=your_private_key” >> .env
echo “API_KEY=your_api_key” >> .env
npm install dotenv
Buying Tron Energy With API: Step-by-Step API Magic
Here’s where you save big. Using Tronsave’s Tron energy API, you can buy energy in seconds, cutting TRC20 gas fees like a pro. We’ll cover a TronWeb script, a Postman shortcut, and error fixes. This is your cheat code to cheap USDT transfers.
TronWeb Script
This code buys 1M energy for 1 hour with Tronsave:
javascript
const TronWeb = require(‘tronweb’)
const TRONSAVE_RECEIVER_ADDRESS = “TWZEhq5JuUVvGtutNgnRBATbF8BnHGyn4S” //in testnet mode change it to “TATT1UzHRikft98bRFqApFTsaSw73ycfoS”
const PRIVATE_KEY = “your_private_key” //Change it
const TRON_FULLNODE = “https://api.trongrid.io” //in testnet mode change it to “https://api.nileex.io”
const TRONSAVE_API_URL = “https://api.tronsave.io” //in testnet mode change it to “https://api-dev.tronsave.io”
const REQUEST_ADDRESS = “your_request_address” //Change it
const TARGET_ADDRESS = “your_target_address” //Change it
const BUY_AMOUNT = 100000 //Chanageable
const DURATION = 3 * 86400 * 1000 //3 days.Changeable
const tronWeb = new TronWeb({
fullNode: TRON_FULLNODE,
solidityNode: TRON_FULLNODE,
eventServer: TRON_FULLNODE,
privateKey: PRIVATE_KEY,
})
const GetEstimate = async (request_address, target_address, amount, duration) => {
const url = TRONSAVE_API_URL + “/v0/estimate-trx”
//see more at https://docs.tronsave.io/buy-energy-on-telegram/using-api-key-to/buy-energy
const body = {
“amount”: amount,
“buy_energy_type”: “MEDIUM”,
“duration_millisec”: duration,
“request_address”: request_address,
“target_address”: target_address || request_address,
“is_partial”: true
}
const data = await fetch(url, {
method: “POST”,
headers: {
“content-type”: “application/json”,
},
body: JSON.stringify(body)
})
const response = await data.json()
return response
}
const GetSignedTransaction = async (estimate_trx, request_address) => {
const dataSendTrx = await tronWeb.transactionBuilder.sendTrx(TRONSAVE_RECEIVER_ADDRESS, estimate_trx, request_address)
const signed_tx = await tronWeb.trx.sign(dataSendTrx, PRIVATE_KEY);
return signed_tx
}
const CreateOrder = async (signed_tx, target_address, unit_price, duration) => {
const url = TRONSAVE_API_URL + “/v0/buy-energy”
//see more at https://docs.tronsave.io/buy-energy-on-telegram/using-api-key-to/buy-energy
const body = {
“resource_type”: “ENERGY”,
“unit_price”: unit_price,
“allow_partial_fill”: true,
“target_address”: target_address,
“duration_millisec”: duration,
“tx_id”: signed_tx.txID,
“signed_tx”: signed_tx
}
const data = await fetch(url, {
method: “POST”,
headers: {
“content-type”: “application/json”,
},
body: JSON.stringify(body)
})
const response = await data.text()
return response
}
const BuyEnergyUsingPrivateKey = async () => {
//Step 1: Estimate the cost of buy order
const estimate_data = await GetEstimate(REQUEST_ADDRESS, TARGET_ADDRESS, BUY_AMOUNT, DURATION)
const { unit_price, estimate_trx, available_energy } = estimate_data
console.log(estimate_data)
const is_ready_fulfilled = available_energy >= BUY_AMOUNT //if available_energy equal buy_amount it means that your order can be fulfilled 100%
if (is_ready_fulfilled) {
//Step 2: Build signed transaction by using private key
const signed_tx = await GetSignedTransaction(estimate_trx, REQUEST_ADDRESS)
console.log(signed_tx);
//Step 3: Create order
const dataCreateOrder = await CreateOrder(signed_tx, TARGET_ADDRESS, unit_price, DURATION)
console.log(dataCreateOrder);
}
}
BuyEnergyUsingPrivateKey()
Postman Quick Test No coding? Use Tronsave’s Postman collection:
- Import at docs.tronsave.io/postman.
- Add your API key from docs.tronsave.io/developer/get-api-key.
- Send POST /buy-energy:
json
{“amount”: 1000000, “duration”: 3600}
- Check the txid in the response.
Error Fixes
- Insufficient TRX: Check balance with tronWeb.trx.getBalance.
- Rate Limits: Tronsave caps at 100 requests/hour—add retry logic.
- Invalid Address: Verify at docs.tronsave.io.
Keep It Secure with Tronsave
Automation is powerful, but one slip can hurt. I once exposed a private key on GitHub—yep, rookie move. Tronsave, with its TRON Stake 2.0 security, keeps your Tron energy exchange safe. Here’s how:
- Secure Keys: Use AWS KMS or encrypt .env files:
javascript
const AWS = require(‘aws-sdk’);
const kms = new AWS.KMS();
async function getKey() {
const decrypted = await kms.decrypt({ CiphertextBlob: Buffer.from(process.env.ENCRYPTED_KEY, ‘base64’) }).promise();
return decrypted.Plaintext.toString();
}
- Avoid API Abuse: Monitor with rate-limiter-flexible (npm install rate-limiter-flexible):
- javascript
const { RateLimiterMemory } = require(‘rate-limiter-flexible’);
const limiter = new RateLimiterMemory({ points: 100, duration: 3600 });
await limiter.consume(‘api_key’);
- Audit Transactions: Check energy on Tronscan:
javascript
const account = await tronWeb.trx.getAccount(tronWeb.defaultAddress.base58);
console.log(‘Energy:’, account.energy);
Share your security hacks on r/Tronix—I’m all ears!
FAQs and Troubleshooting
Hit a snag? Here’s how to fix common Tron energy API issues, plus answers from the Tron community.
FAQs:
- Why’s my transaction failing? Likely low TRX or energy. Check with tronWeb.trx.getBalance.
- How to activate an account? Send 0.1 TRX via tronWeb.trx.sendTrx.
- Best Tron energy calculator? Use Tronsave’s embedded tool below or /estimate-trx endpoint.
Troubleshooting:
- Insufficient Energy: Buy more via Tronsave or try Tron energy sell platforms.
- Rate Limit Hit: Wait or switch providers. Add retries.
- Tx Not Found: Verify txid on Tronscan.
Drop questions in our Q&A widget [embedded widget]—we’ve got you.
Table: Fix It Fast
Error | Cause | Solution |
Insufficient Energy | Low energy | Buy via Tronsave API |
Rate Limit Exceeded | Too many requests | Retry logic, backup provider |
Invalid Address | Wrong address | Check docs.tronsave.io |
The Bottom Line: Save Big with Tronsave
High TRC20 gas fees don’t have to haunt your dApp. With Tronsave’s Tron energy API, you can cut USDT TRC20 transaction fees by 80-90%, scale effortlessly, and even earn passive income via staking (4-6% APR). Built on TRON Stake 2.0, Tronsave—proud Top 3 in Tron Hackathon Season 4 and Season 5 Builder winner—offers unmatched security and reliability. Whether you’re a dev or a DeFi pro, this guide gives you the code, tools, and know-how to thrive in Tron’s 2025 ecosystem.
Ready to slash fees and boost your dApp? Buy energy now at docs.tronsave.io/developer/get-api-key or join the Tron community on r/Tronix to share your savings. Let’s build the Web 3 future together!